In this tutorial, we will be creating an HTML page that can toggle between light and dark theme using Bootstrap 5 and JavaScript.
Prerequisites
- A text editor
- A modern web browser
Writing the HTML
Firstly, we need to create the HTML skeleton for our page. This will include the basic structure, the Bootstrap 5 stylesheet, the necessary JavaScript libraries and the necessary Bootstrap 5 classes.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Bootstrap Toggle Demo</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
</head>
<body class="light">
<div class="container">
<button type="button" class="btn btn-primary" data-toggle="light">Light Theme</button>
<button type="button" class="btn btn-primary" data-toggle="dark">Dark Theme</button>
</div>
</body>
</html>
Writing the JavaScript Logic
Next, we need to add some JavaScript to our page. This will handle the toggling of the theme.
$(function(){
$('.btn-primary').on('click', function(){
var theme = $(this).data('toggle');
if (theme === 'light') {
$('body').addClass('light');
$('body').removeClass('dark');
} else {
$('body').addClass('dark');
$('body').removeClass('light');
}
});
});
The code $('.btn-primary').on('click', function(){})
is a jQuery selector which is used to bind a function to the click event of all elements with the class .btn-primary
.
The code var theme = $(this).data('toggle');
is used to store the value of the data-toggle attribute of the clicked element in the theme variable. The code if (theme === 'light')
is used to check if the theme variable has the value light. If it does, then the code block within the curly braces will be executed. The code block within the curly braces will add the class light to the body element and remove the class dark. The code else
is used to check if the theme variable has the value dark. If it does, then the code block within the curly braces will be executed. The code block within the curly braces will add the class dark to the body element and remove the class light.
Writing the Stylesheet
Then, we will add light and dark to the style classes for our body element inside our HTML head tags.
<style>
body.light {
background-color: #f5f5f5;
color: black;
}
body.dark {
background-color: #222;
color: white;
}
</style>
The body.light
css selector sets the background-color to #f5f5f5 and the color to black. The body.dark
css selector sets the background-color
to #222
and the color
to white
.
Our result:
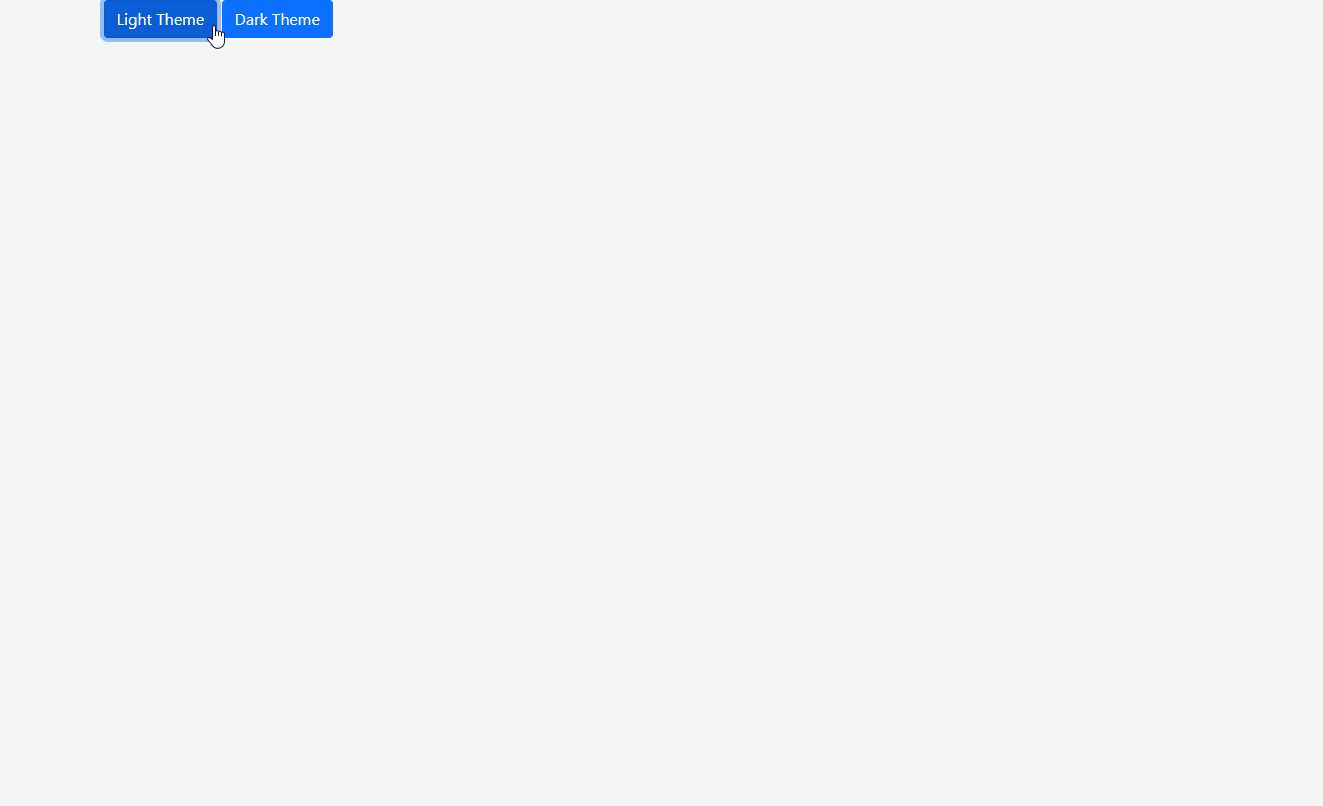
Conclusion
That’s it! We have now created an HTML page that can toggle between light and dark theme using Bootstrap 5 and JavaScript.